Competition Code
Formatting Code for the Vex Robotics Competition
A template with the function callbacks for autonomous and usercontrol mode is required to perform in a vex competition. When you are at a competition you connect your robot to the field controller that will start and stop your code based on timer and mode of the match. If you code is not in this template it will not run properly.
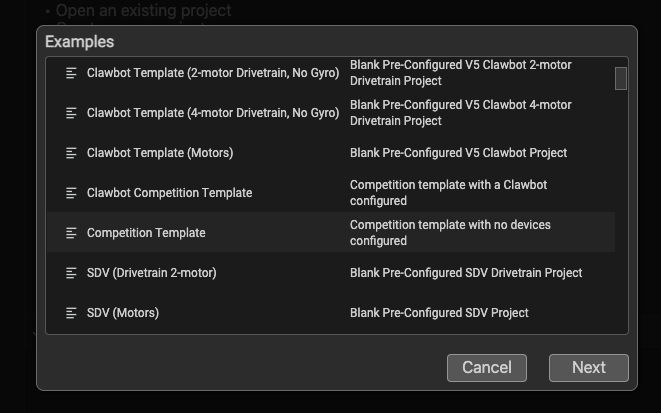
A competition Template is available in the vex code IDE. Choose File/Open Example. and you will get the above window, scroll to find the Competition Template then click next you will need to give it a unique project name.
Competition Template
Here is the code that is in main.cpp you can open a new project and copy paste this code to start or you cold find this code from the example codes that VexCode offers.
NOTE: The competition template has three sections that correspond to the three phases of a competition match: Pre Autonomous (robot setup), Autonomous period, and Driver Controlled Period.
In order for your code to work at a competition, you must:
- Leave the code below inside the main function in place.
- Add your code inside one of the three function (
pre_auton
,autonomous
,usercontrol
).
Main is the code that runs when you start the code
int main() { // Set up callbacks for autonomous and driver control periods. Competition.autonomous(auton); Competition.drivercontrol(driver); // Run the pre-autonomous function. pre_auton(); // Prevent main from exiting with an infinite loop. while (true) { wait(100, msec); } }
Using a Pre-auton Function for Any Setup Steps
Add any setup steps such as gyro calibration, or other sensor resets that should run when the program is started, to the pre_auton
function.
NOTE: The code inside the pre_auton
function below will run immediately when the program is started, before the autonomous portion of the match begins.
NOTE: If you choose not to use this section, leave it empty.
Using the Autonomous Function for Any Autonomous Routine
Put the code for your autonomous routine into the autonomous
function. Your autonomous
function must only contain commands that won’t need any interaction from a user. (E.g., BumperA.pressing()
)You should avoid any commands that require a user’s interaction. E.g Controller1.ButtonA.pressing();
NOTE: You can copy and paste this code from another project file.
Using the usercontrol Function for Any User Controlled Routines that Take Place During the Driver Controlled Period
The usercontrol
function must only contain commands controlled by the user. (E.g. Controller1.Axis1.position();
)Put your driver control code into the usercontrol
function, inside the while(1)
loop and before the wait(20, msec)
command.
/*----------------------------------------------------------------------------*/ /* */ /* Module: main.cpp */ /* Author: VEX */ /* Created: Thu Sep 26 2019 */ /* Description: Competition Template */ /* Update: DK 6-11-2021 */ /*----------------------------------------------------------------------------*/ // ---- START VEXCODE CONFIGURED DEVICES ---- // Robot Configuration: // [Name] [Type] [Port(s)] // Controller1 controller // ---- END VEXCODE CONFIGURED DEVICES ---- #include "vex.h" using namespace vex; // A global instance of competition competition Competition; // define your global Variables here float pi=3.14; // Custom Functions void pre_auton() { // Initializing Robot Configuration. DO NOT REMOVE! vexcodeInit(); // All activities that occur before the competition starts // Example: clearing encoders, setting initial positions, ... } void auton() { } void driver() { // User control code here, inside the loop while (true) { wait(10, msec); // Sleep the task for a short amount of time to prevent wasted resources. } } int main() { // Set up callbacks for autonomous and driver control periods. Competition.autonomous(auton); Competition.drivercontrol(driver); // Run the pre-autonomous function. pre_auton(); // Prevent main from exiting with an infinite loop. while (true) { wait(100, msec); } }
Using the Remote to Start Competition Code
The code to run and the mode to run it in can be selected by the remote. You have 3 choices, simply run the code, run in timed mode, or competition mode.
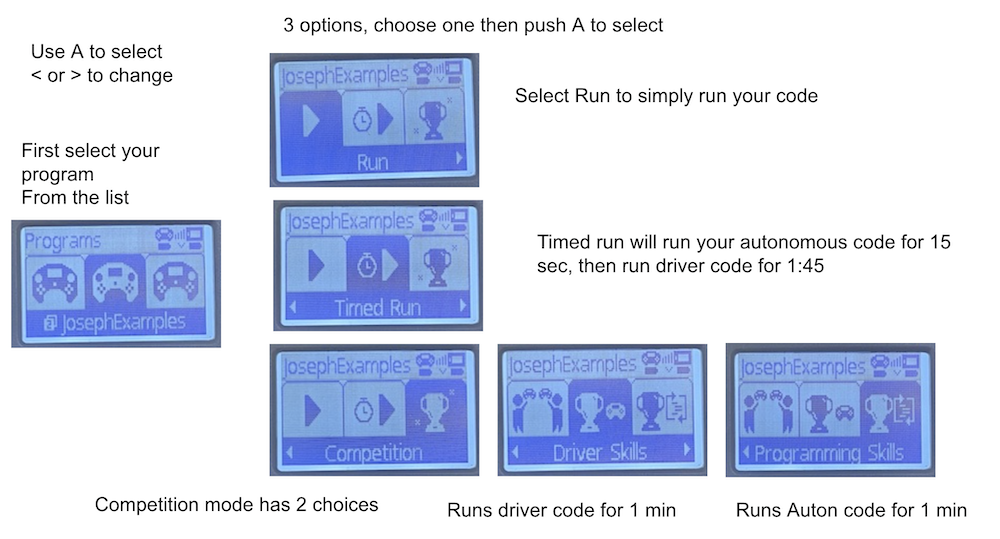
With your code written in the competition template you can simulate real matches with a timer.