V5 GUI for Auton Selector
Screen Interface to Select Auton
Typically a robot will have several options for the autonomous mode. The mode needs to be selected before a match. Here we will implement a Graphical User Interface or GUI to make this selection. There are a few new concepts used in this code:
- drawRectangle method to draw buttons on the screen
- Event driven code using a function callback when the screen is touched
- Brain.Screen.xPosition() and yPosition() to get the position of the screen touch
This is all put together to increment a variable that is later used in a switch case structure to runs the desired autonomous routine.
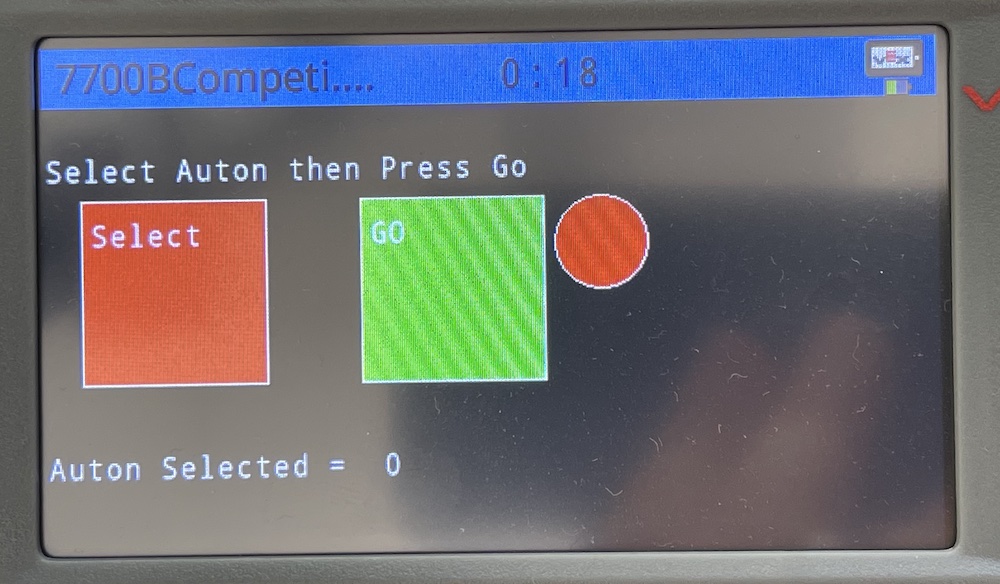
We will be creating a screen that looks like this. The red Select button will be used to increment the value of autonSelect variable, the Green GO button will set a flag indicating that auton has been selected.
Code for Select
First we set up some global variables to be used for auton select.
int AutonSelected = 0; int AutonMin = 0; int AutonMax = 4;
autonSelect will be used in the switch case to run the correct auton. autonMin and autonMax are the minimum and maximum values we want for autonSelect. it may be that you have autons 1,2,3,4. so autonMin=1 and autonMax=4 or maybe 0,1,2,3. autonMin=0 and autonMax=3. etc.
Code for the GUI
The following code draws the GUI. This only needs to run once to draw the buttons and instructions on the screen. Call this function from preAuton().
void drawGUI() { // Draws 2 buttons to be used for selecting auto Brain.Screen.clearScreen(); Brain.Screen.printAt(1, 40, "Select Auton then Press Go"); Brain.Screen.printAt(1, 200, "Auton Selected = %d ", autonSelect); Brain.Screen.setFillColor(red); Brain.Screen.drawRectangle(20, 50, 100, 100); Brain.Screen.drawCircle(300, 75, 25); Brain.Screen.printAt(25, 75, "Select"); Brain.Screen.setFillColor(green); Brain.Screen.drawRectangle(170, 50, 100, 100); Brain.Screen.printAt(175, 75, "GO"); Brain.Screen.setFillColor(black); }
The code just draws a couple rectangles on the screen and labels them. there is also a signal shown by drawing a circle Brain.Screen.drawCircle(300, 75, 25); that will be red until you press GO. This should help the user not forget to select the auton.
Since we are changing fill color at the end we have a clean up that resets the fillColor to black. Here we should also make any other resets of things that were changed like font size, penColor etc.
Code for Selecting
Here is code that will get the value of where the screen was pressed and decide what to do . The method Brain.Screen.xPosition() will return the x position of the last touch on the screen , similarly .yposition() returns the y value. We then have to decide which button was pressed. This is done with a set of AND checks. for an AND to be true both sides of the AND must be true. So
(x >= 20 && x <= 120)
is TRUE only when x is both >=20 and <=120. This represents the two sides of the button. Similar check for y.
When the if condition is true we need to increment the value of autonSelect
AutonSelected++;
adds one to the value of autonSelect.
Rollover
We only want to count up to autonMax, so we need some code to roll back to autonMin when we go above the max.
if (AutonSelected > AutonMax)AutonSelected = AutonMin; // rollover
will set autonSelect back to autonMin when we go over.
We also need a signal to know that we are done selecting this is done using the flag selectingAuton. it is first set to true and changed to false if the last touch was the GO button. When it is TRUE we change the color of the signal circle to green.
if (!selectingAuton) { Brain.Screen.setFillColor(green); Brain.Screen.drawCircle(300, 75, 25); }
This can be seen from a distance as a simple check that we are done selecting auton.
Here is the complete function
void selectAuton() { bool selectingAuton = true; int x = Brain.Screen.xPosition(); // get the x position of last touch of the screen int y = Brain.Screen.yPosition(); // get the y position of last touch of the screen // check to see if buttons were pressed if (x >= 20 && x <= 120 && y >= 50 && y <= 150) // select button pressed { AutonSelected++; if (AutonSelected > AutonMax)AutonSelected = AutonMin; // rollover Brain.Screen.printAt(1, 200, "Auton Selected = %d ", AutonSelected); } if (x >= 170 && x <= 270 && y >= 50 && y <= 150) { selectingAuton = false; // GO button pressed Brain.Screen.printAt(1, 200, "Auton = %d GO ", AutonSelected); } if (!selectingAuton) { Brain.Screen.setFillColor(green); Brain.Screen.drawCircle(300, 75, 25); } else { Brain.Screen.setFillColor(red); Brain.Screen.drawCircle(300, 75, 25); } wait(10, msec); // slow it down Brain.Screen.setFillColor(black); }
Lastly we have a 10msec wait to slow things down for human response time and we do a clean up to put the fill color back to black.
The Function Callback
We will implement a function callback to run the selectAuton function. A function callback is triggered by an EVENT here the event is a touch on the screen. Once we write a function callback it can be used over and over each time the EVENT occurs. We write this event in preAuton. Note the callback function can not have anything passed to it and the call only requires the name of the function.
Brain.Screen.pressed(selectAuton);
Sets the callback to selectAuton. Each time the screen is pressed the function will run once. It will only run again until after the screen press is released and pressed again. This way we get only one increment per press.
Putting it All Together
We need to set up the GUI and call selectAuton from the preAuton function. The use the result in a switch case in the auton function.
void pre_auton() { // Initializing Robot Configuration. DO NOT REMOVE! vexcodeInit(); Brain.Screen.printAt(1, 40, "pre auton is running"); drawGUI(); Brain.Screen.pressed(selectAuton); } void auton() { switch (AutonSelected) { case 0: //code 0 break; case 1: //code 1 break; case 2: //code 2 break; case 3: //code 3 break; } }
Try It Yourself
You can type this all from scratch or you can copy and paste. If you copy and paste you must be sure to put things in the right place and keep track of all your code blocks separated by hamburger brackets {}.
Remember functions must be written before they are called
You can personalize it to your team, use your favorite colors, make the labels more descriptive etc. You can use:
Brain.Screen.setFont(monoM);
to change font size.