7-Segment Display
Displaying Numbers with LED Circuits
.
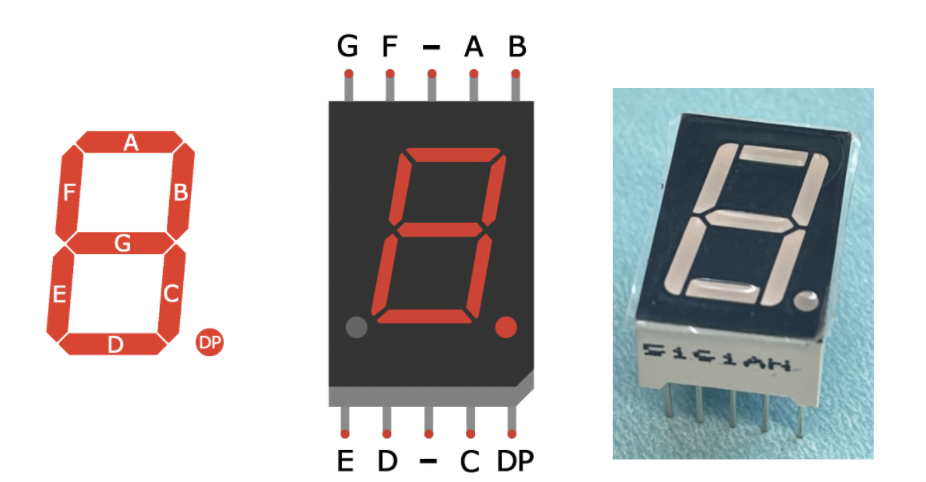
The display is basically eight LEDs in a single package arranged to display a number. Each circuit is the same as out basic LED circuit. We have two pins for - that connect to ground through a resistor and 8 other pins that each connect to their own digital output pin.
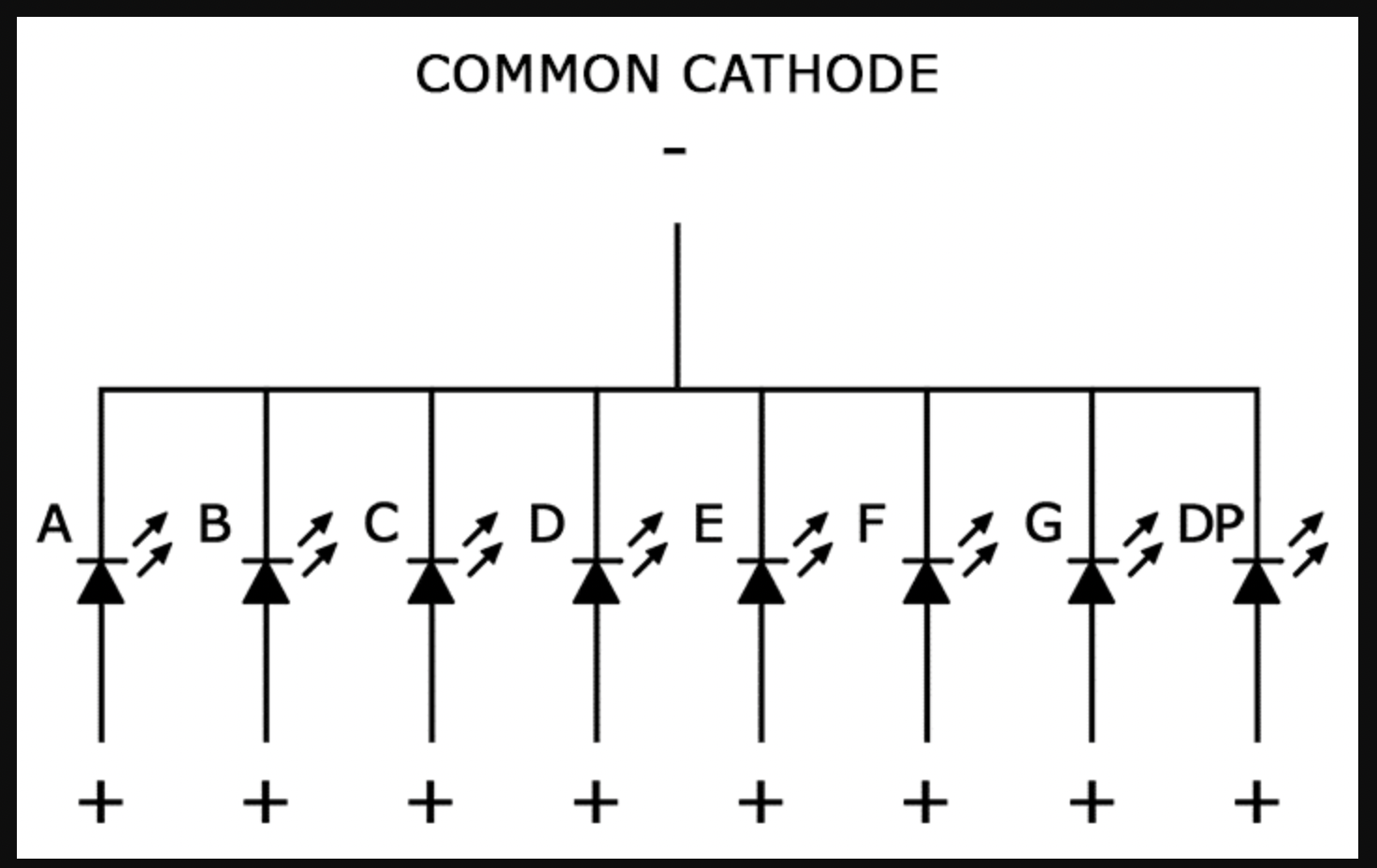
Schematic for the 7 segment display. It actually consists of 8 LEDs, the 7 segments and the decimal point. All are connected together at the negative side, this way we only need one resistor and one connection to GND.
Lets Write Clean Code
In this project we will reuse much of the code from the previous light show lessons. Rather than just copy and paste lets see if we can rewrite most of this in a clean fashion. Clean code should be readable as if it was written in plain english. This will make it much easier for others to read use and develop further.
What is meant by clean code?
- Variable names should mean something
- Functions names should be meaningful and use a verb in their name
- Functions are best if they do only one thing
- Comments should precede each function with a description of what it does
- Use many comments
All of these items will make your code more readable.
Name the Pins
//Set up the pin names for the 7 segment display const int top = 2; //A const int topRight=3;//B const int bottomRight=4;//C const int bottom=5;//D const int bottomLeft=6;//E const int topLeft=7;//F const int middle=8;//G const int decimal=9;//DP
Now that we have these named we dont have to think about the numbers, hopefully these names are more rememberable than the numbers or letters of the pins. With these names we set the pins as digital OUTPUT in the setup function
void setup() { //set the pins as digital OUTPUT pinMode(top, OUTPUT); pinMode(topRight, OUTPUT); pinMode(bottomRight, OUTPUT); pinMode(bottom, OUTPUT); pinMode(bottomLeft, OUTPUT); pinMode(topLeft, OUTPUT); pinMode(middle, OUTPUT); pinMode(decimal, OUTPUT); //Start a serial connection Serial.begin(9600); }
We also set up a serial connection to use for printing to the screen.
Setting the State of the Display
We will write a custom function similar to the lightLEDs function of the previous lessons. That function would control 5 LEDs and set them as on or off. The new function will control the 8 LEDs of the display. It will take 8 boolean arguments to define the state of each segment. If the boolean is 1 the segment is on if it is 0 the segment is off. it is simpler than it looks.
//A function to set the state of every segment of the LED display //The function accepts 8 arguments one for each segment of the display // a value of 1 the segment is on a value of 0 it is off void segmentDisplayState(bool topState, bool topRightState, bool bottomRightState, bool bottomState, bool bottomLeftState, bool topLeftState, bool middleState, bool decimalState) { digitalWrite(top,topState); digitalWrite(topRight,topRightState); digitalWrite(bottomRight,bottomRightState); digitalWrite(bottom, bottomState); digitalWrite(bottomLeft, bottomLeftState); digitalWrite(topLeft, topLeftState); digitalWrite(middle, middleState); digitalWrite(decimal, decimalState); }
In each digitalWrite statement we have the name of the pin something like top and its state something like topState
A call to this function takes this form:
segmentDisplayState(1,1,1,0,0,0,0,0);
This call will light the top, topRight and bottomRight segment, representing the number 7
Display Numbers
We will now write a function to display numbers. We need to set the state of the display to show numbers like 1, 3 5 etc. We will use a switch case. We just need to figure out the calls for specific number. We already know that 7 is displayed by
segmentDisplayState(1,1,1,0,0,0,0,0);
A little thought reveals that the number one is displayed just by lighting the right two segments with the call
segmentDisplayState(0,1,1,0,0,0,0,0);
Here is the basic structure of the function using switch case. See if you can fill in the calls for each number.
//displays a number by setting the state of the display void displayNumber(int number) { switch (number) { case 0: segmentDisplayState(1, 1, 1, 1, 1, 1, 0, 0); break; case 1: segmentDisplayState(0, 1, 1, 0, 0, 0, 0, 0); break; case 2: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; case 3: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; case 4: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; case 5: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; case 6: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; case 7: segmentDisplayState(1, 1, 1, 0, 0, 0, 0, 0); break; case 8: segmentDisplayState(1, 1, 1, 1, 1, 1, 1, 0); break; case 9: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); break; default: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 1); break; break; } }
This function is not complete, you need to adjust the arguments of the segmentDisplayState function call to get the number display correct.
Display a Random Number
Add a function to choose a random number and then display it.
//Function that displays a random number 0 to 9 void displayRandom() { int randomNumber =random(0,10); displayNumber(randomNumber); }
This function is simple because it uses the two previous functions that we have written.
Putting it All Together
Add a couple of test functions, testLEDs and testNumbers. Then in void loop call the random number display function displayRandom()
Example Code
//Set up the pin names for the 7 segment display const int top = 2; //A const int topRight = 3; //B const int bottomRight = 4; //C const int bottom = 5; //D const int bottomLeft = 6; //E const int topLeft = 7; //F const int middle = 8; //G const int decimal = 9; //DP void setup() { //set the pins as digital OUTPUT pinMode(top, OUTPUT); pinMode(topRight, OUTPUT); pinMode(bottomRight, OUTPUT); pinMode(bottom, OUTPUT); pinMode(bottomLeft, OUTPUT); pinMode(topLeft, OUTPUT); pinMode(middle, OUTPUT); pinMode(decimal, OUTPUT); //Start a serial connection Serial.begin(9600); testLEDs(300); testNumbers(1000); } //function to light all the LEDs as a test of the circuit // time is set by the integer value of t so you can make it faster or slower void testLEDs(int t) { segmentDisplayState(1, 0, 0, 0, 0, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 0, 0, 0, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 0, 0, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 0, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 1, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 1, 1, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 1, 1, 1, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 1, 1, 1, 1); delay(t); segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); delay(t); segmentDisplayState(1, 1, 1, 1, 1, 1, 1, 1); delay(t); segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 0); delay(t); } void testNumbers(int t) { for (int i = 0; i <= 10; i++) { displayNumber(i); delay(t); } } //A function to set the state of every segment of the LED display //The function accepts 8 arguments one for each segment of the display // a value of 1 the segment is on a value of 0 it is off void segmentDisplayState(bool topState, bool topRightState, bool bottomRightState, bool bottomState, bool bottomLeftState, bool topLeftState, bool middleState, bool decimalState) { digitalWrite(top, topState); digitalWrite(topRight, topRightState); digitalWrite(bottomRight, bottomRightState); digitalWrite(bottom, bottomState); digitalWrite(bottomLeft, bottomLeftState); digitalWrite(topLeft, topLeftState); digitalWrite(middle, middleState); digitalWrite(decimal, decimalState); } //displays a number by setting the state of the display void displayNumber(int number) { switch (number) { case 0: segmentDisplayState(1, 1, 1, 1, 1, 1, 0, 0); break; case 1: segmentDisplayState(0, 1, 1, 0, 0, 0, 0, 0); break; case 2: segmentDisplayState(1, 1, 0, 1, 1, 0, 1, 0); break; case 3: segmentDisplayState(1, 1, 1, 1, 0, 0, 1, 0); break; case 4: segmentDisplayState(0, 1, 1, 0, 0, 1, 1, 0); break; case 5: segmentDisplayState(1, 0, 1, 1, 0, 1, 1, 0); break; case 6: segmentDisplayState(0, 0, 1, 1, 1, 1, 1, 0); break; case 7: segmentDisplayState(1, 1, 1, 0, 0, 0, 0, 0); break; case 8: segmentDisplayState(1, 1, 1, 1, 1, 1, 1, 0); break; case 9: segmentDisplayState(1, 1, 1, 0, 0, 1, 1, 0); break; default: segmentDisplayState(0, 0, 0, 0, 0, 0, 0, 1); break; break; } } //Function that displays a random number 0 to 9 void displayRandom() { int randomNumber = random(0, 10); displayNumber(randomNumber); } void loop() { displayRandom(); delay(1000); }