Flora Adafruit
Flora Adafruit
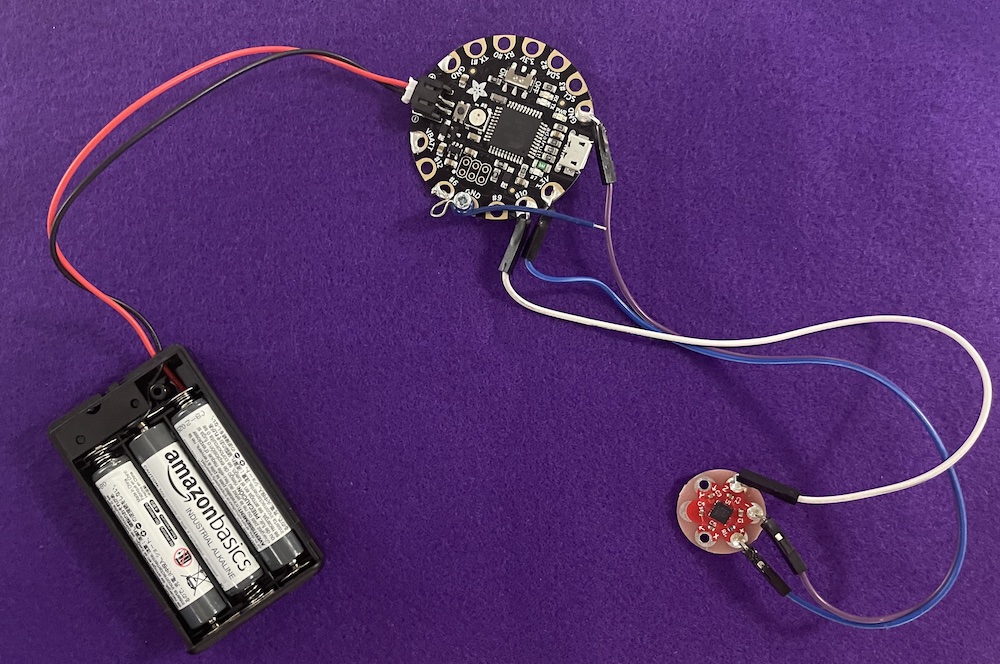
Battery, Flora, Accellerometer
AdaFruit Flora
here is some basic code to get started with the Adafruit Flora device. It combines the blink LED and display colors on NEO Pixel. In this code we blink the standard LED on pin 7 3 times to indicate that our set up is complete, then proceed to walk the NEOpixel through several colors.
#include#define PIN 8 // Pin D7 has an LED connected on FLORA. // give it a name: int led = 7; Adafruit_NeoPixel strip = Adafruit_NeoPixel(1, PIN, NEO_GRB + NEO_KHZ800); void setup() { // initialize the digital pin as an output. pinMode(led, OUTPUT); strip.begin(); strip.setBrightness(50); strip.show(); // Initialize all pixels to 'off' //blink the led to indicate set up is done for (int i = 0; i <= 3; i++) { digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level) delay(1000); // wait for a second digitalWrite(led, LOW); // turn the LED off by making the voltage LOW delay(1000); // wait for a second } } void loop() { // Some example procedures showing how to display to the pixels: colorWipe(strip.Color(255, 0, 0), 500); // Red colorWipe(strip.Color(0, 255, 0), 500); // Green colorWipe(strip.Color(0, 0, 255), 500); // Blue rainbowCycle(20); } // Fill the dots one after the other with a color void colorWipe(uint32_t c, uint8_t wait) { for(uint16_t i=0; i
Accelerometer
code to read and print values of an analog accelerometer ADXL345
//code to read analog accelerometer // Pin D7 has an LED connected on FLORA. // give it a name: int led = 7; void setup() { Serial.begin(9600); // initialize the digital pin as an output. pinMode(led, OUTPUT); //blink the led to indicate set up is done for (int i = 0; i <= 3; i++) { digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level) delay(1000); // wait for a second digitalWrite(led, LOW); // turn the LED off by making the voltage LOW delay(1000); // wait for a second } } int a=0; int out=0; void loop() { a=analogRead(10); out=map(a,400,700,0,255); Serial.print("out= "); Serial.print(out); Serial.print(" accell= "); Serial.println(a); delay(200); }
Acceleration to Colors
One example of using the accelerometer. Here the value of acceleration in Z direction is used to change the color of the neopixel LED.
//code to read analog accelerometer and show colors #include#define PIN 8 Adafruit_NeoPixel strip = Adafruit_NeoPixel(1, PIN, NEO_GRB + NEO_KHZ800); // Pin D7 has an LED connected on FLORA. // give it a name: int led = 7; void setup() { Serial.begin(9600); // initialize the digital pin as an output. pinMode(led, OUTPUT); strip.begin(); strip.setBrightness(50); strip.show(); // Initialize all pixels to 'off' //blink the led to indicate set up is done for (int i = 0; i <= 3; i++) { digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level) delay(1000); // wait for a second digitalWrite(led, LOW); // turn the LED off by making the voltage LOW delay(1000); // wait for a second } } int a=0; int out=0; void loop() { a=analogRead(10); out=map(a,400,700,0,255); Serial.print("out= "); Serial.print(out); Serial.print(" accell= "); Serial.println(a); colorWipe(strip.Color(out, 0, 255-out), 500); } // Fill the dots one after the other with a color void colorWipe(uint32_t c, uint8_t wait) { for(uint16_t i=0; i
Back to the course
Lessons
Making Noise
2001